-
Notifications
You must be signed in to change notification settings - Fork 1
Design and Planning
Rev. 1.0 2022-10-22 - initial version
Rev. 1.1 2022-11-21 - Modify API Spec (For Successful Quality Pass)
Rev. 1.2 2022-11-26 - Modify Architecture Figure (Redux -> Zustand)

We use AWS EC2 as a server and AWS S3 for storing documents that are uploaded through our service. Nginx http server is used because it is light and fast web server and can be utilized for reverse proxy. To establish our system safe and easy to scale up, building docker images of frontend & backend would be a good option. We use Guincorn to handle simultaneous backend request instead of uWSGI. We chose Flux for design pattern of frontend, so React and Redux will form Dispatcher-Action-View structure.
- Using Ant-Design, dx-react-scheduler, react-dnd libraries.
- Solid lines for link, navigation, relationships between components and dotted lines for subcomponent relationships.
- Component 'Menu' is a subcomponent to the others, so for the convenience, dotted lines are ommited which start from 'Menu' component.
- UserState
- email, password, phoneNumber, projects: ProjectState[]
- ProjectState
- name, subject, users: UserState[]
- MeetingState
- project: ProjectState, meetAt, content
- TaskState
- name, project: ProjectState, content, assignee: UserState, createdAt, updatedAt, untilAt
- CommentState
- user: UserState, task: TaskState, content
- ReactionState
- user: UserState, comment: CommentState, emoji
- DocumentState
- name, document, createdAt, head: boolean
- DocumentSpaceState
- name, project: ProjectState, documents: DocumentState[]
- TimeTableState
- user: UserState, schedule
- NotificationState
- user: UserState, content, checked: boolean
Slice | Action | Function |
---|---|---|
User | signUp(email, name, password) | sign up a new user |
login(email, password) | login user | |
logout() | logout user | |
editUser(userId) | edit user information | |
deleteUser(userId) | delete user | |
Project | getProject(userId) | get user-involved projects |
createProject(project: ProjectState) | create new project | |
editProject(projectId) | edit project | |
deleteProject(projectId) | delete project | |
Meeting | getMeeting(projectId) | get meetings under a project |
createMeeting(meeting: MeetingState) | create new meeting | |
editMeeting(meetingId) | edit meeting | |
deleteMeeting(meetingId) | delete meeting | |
Task | getTask(projectId) | get tasks under a project |
createTask(task: taskState) | create new task | |
editTask(taskId) | edit task | |
deleteTask(taskId) | delete task | |
Comment | getComment(taskId) | get comments under a task |
createComment(comment: CommentState) | create new comment | |
editComment(commentId) | edit comment | |
deleteComment(commentId) | delete comment | |
Reaction | getReaction(commentId) | get reactions under a comment |
createReaction(reaction: ReactionState) | create new reaction | |
editReaction(reactionId) | edit reaction | |
deleteReaction(reactionId) | delete reaction | |
Document | getDocument(documentSpaceId) | get documents under a document space |
createDocument(document) | create new document | |
DocumentSpace | getDocumentSpace(projectId) | get document spaces under a project |
createDocumentSpace(documentSpace) | create new document space | |
editDocumentSpace(documentSpaceId) | edit document space | |
deleteDocumentSpace(documentSpaceId) | delete document space |
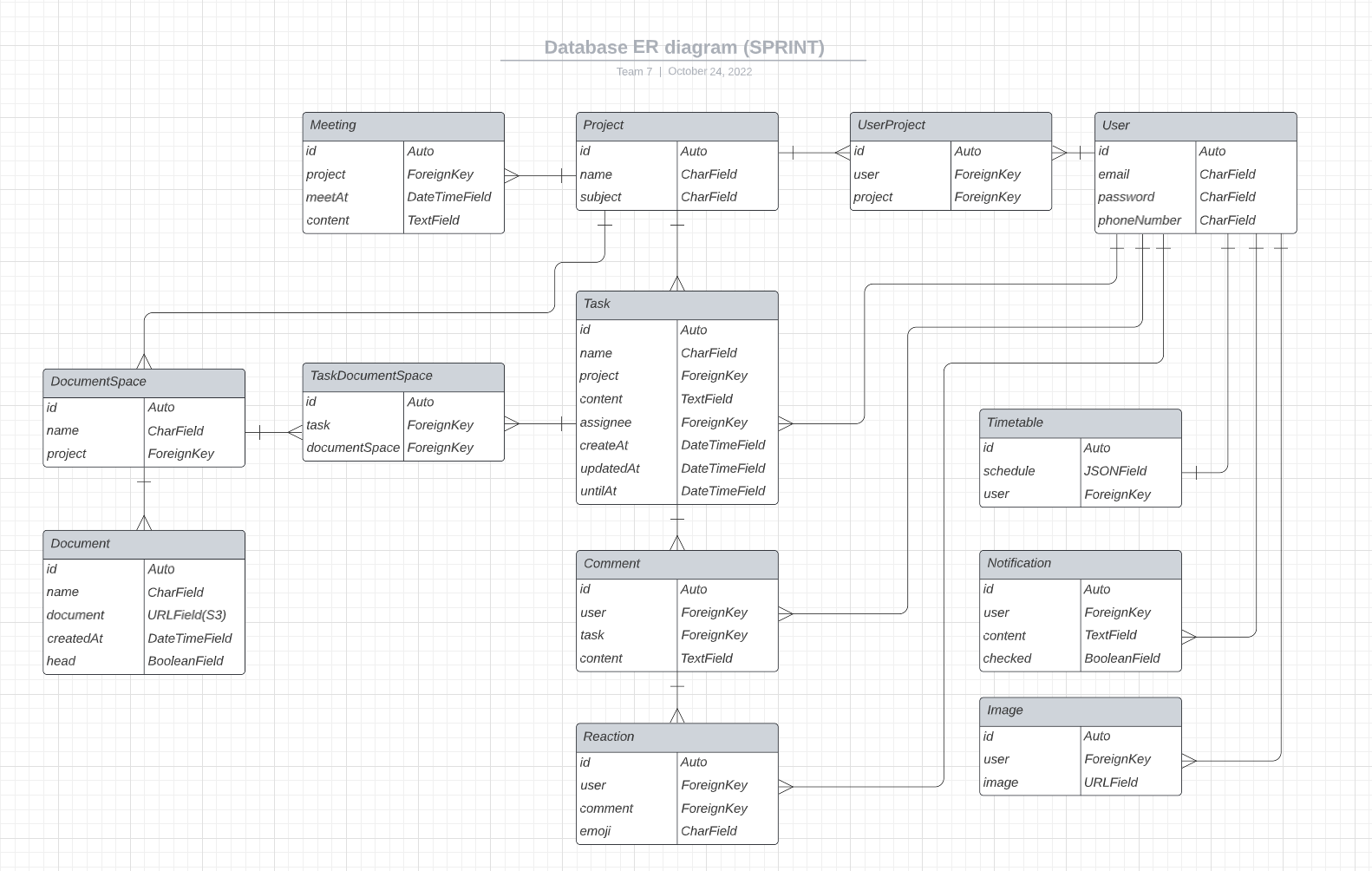
ManyToManyField in Django is converted into a NEW entity and two OneToMany relation automatically. Therefore we drew additional entities and relations to get clarity. For scalability, User entity has simple attributes but entities about user profile are connected to User. Although we didn't mark created time on every entity, it will be included because it can be useful to manage database.
Model | API | GET | POST | PUT | DELETE |
---|---|---|---|---|---|
User | /api/user/signup/ | X | Create new user | X | X |
/api/user/signin/ | X | Log in | X | X | |
/api/user/signout/ | Log out | X | X | X | |
/api/user/change/ | X | X | Change user settings | Delete user | |
/api/user/info/:user_id/ | Get user info (profile) | X | X | X | |
Timetable | /api/user/timetable/:user_id/ | Get timetable of the user | X | X | X |
/api/user/timetable/:user_id/m/ | X | Create timetable | Change timetable | X | |
Notification | /api/user/noti/:user_id/ | Get all notifications of the user | X | X | X |
/api/user/noti/:user_id/m/ | X | Add notification to the user | X | X | |
/api/user/noti/detail/:noti_id/ | Get notification detail | X | X | X | |
/api/user/noti/detail/:noti_id/m/ | X | X | Change status of the notification | Delete the notification | |
Image | /api/user/image/:user_id/ | Get image of user | X | X | X |
/api/user/image/:user_id/m/ | X | Set user image | Change user image | Delete user image | |
Project | /api/project/:user_id/ | Get project list of the user | X | X | X |
/api/project/:user_id/m/ | X | Create new project | X | X | |
/api/project/detail/:project_id/ | Get project detail | X | X | X | |
/api/project/detail/:project_id/m/ | X | X | Change project detail | Delete project | |
/api/project/member/ | Get all members of the project | X | X | X | |
/api/project/member/m/ | X | X | Add or Subtract member | X | |
Meeting | /api/meeting/:project_id/ | Get meeting list of the project | X | X | X |
/api/meeting/:project_id/m/ | X | Create new meeting | X | X | |
/api/meeting/detail/:meeting_id/ | Get meeting detail | X | X | X | |
/api/meeting/detail/:meeting_id/m/ | X | X | Change meeting detail | Delete meeting | |
Task | /api/task/:project_id/ | Get task list of project | X | X | X |
/api/task/:project_id/m/ | X | Create new task | X | X | |
/api/task/detail/:task_id/ | Get task detail | X | X | X | |
/api/task/detail/:task_id/m/ | X | X | Change task detail (like Assignee) | Delete task | |
/api/task/belong/:user_id/ | Get task list of user | X | X | X | |
/api/task/document/:task_id/ | Get document space list of the task | X | X | X | |
/api/task/document/:task_id/m/ | X | Connect document space and task | X | Disconnect document space and task | |
DocumentSpace | /api/document/:project_id/ | Get document space list of project | X | X | X |
/api/document/:project_id/m/ | X | Create new document space | X | X | |
/api/document/detail/:docuspace_id/ | Get document space detail | X | X | X | |
/api/document/detail/:docuspace_id/m/ | X | X | Change document space detail | Delete document space | |
Document | /api/documents/:docuspace_id/ | Get document list of the document space | X | X | X |
/api/documents/:docuspace_id/m/ | X | Add new document to the space | X | X | |
/api/documents/detail/:document_id/ | Get document detail | X | X | X | |
/api/documents/detail/:document_id/m/ | X | X | Change document detail (like HEAD) | Delete document | |
Comment | /api/comment/:task_id/ | Get comment list of the task | X | X | X |
/api/comment/:task_id/m/ | X | Create new comment to the task | X | X | |
/api/comment/detail/:comment_id/ | Get comment detail | X | X | X | |
/api/comment/detail/:comment_id/m/ | X | X | Change comment content | Delete comment | |
Reaction | /api/reaction/:comment_id/ | Get reaction list of the comment | X | X | X |
/api/reaction/:comment_id/m/ | X | Create new reaction to the comment | X | X | |
/api/reaction/detail/:reaction_id/ | Get reaction detail | X | X | X | |
/api/reaction/detail/:reaction_id/m/ | X | X | Change reaction | Delete reaction |
Page | Tasks | Priority | Time | Sprint | Assignee | Finished |
---|---|---|---|---|---|---|
Log In | User login & redirect to main page | low | 1day | |||
Log Out | User logout | low | 1day | 5 | ||
Sign Up | Create new user | medium | 1day | 5 | ||
My Page | Show user's profile | medium | 2day | |||
Profile edit page | Let user edit his or her profile | medium | 2day | |||
Let user update their timetable | medium | 2day | ||||
Main Page | List up all the projects belog to the user | high | 1day | 3 | 김상훈 최석우 |
|
List up all the notifications | medium | 1day | 4 | |||
ComposeProject Page | Create new project | very high | 2day | 3 | 최석우 김상훈 |
|
Send Email to the team members | medium | 1day | 4 | |||
Project Main Page | Show project information and task list | high | 1day | 3 | 김상훈 최석우 |
|
Show Contribution Summary as a graph | high | 2day | 4 | |||
Project Management Page | Let user edit the project info | medium | 1day | |||
Project Calendar | Show the task deadline and team meeting schedule | medium | 1day | 4 | 최석우 김상훈 |
|
Create Project Meeting | Create new team meeting | medium | 1day | 4 | ||
Create Task | Create new task | very high | 2day | 3 | 주형진 이상현 |
|
Create new document | very high | 2day | 3 | 이상현 주형진 |
||
Document List | List up all the documents | medium | 1day | |||
Document detail | List up all the version changes of the document | medium | 1day | |||
Task View and Edit | Show task information | very high | 2day | 3 | 주형진 이상현 |
|
Upload document | high | 2day | 3 | 이상현 주형진 |
||
Let user leave the comment | medium | 1day | 4 |
- Frontend : Jest & Enzyme
- Backend : Default Python Unit Test
- We aim for code coverage to be >80%.
- Every components should be tested.
- Every components will be tested just after it is created.
- Frontend : Jest & Enzyme
- Backend : Default Python Unit Test
- We aim for code coverage to be >80%.
- If needed, we will mock data to emulate database(MySQL) behavior.
- We will use Travis CI for integration testing.